I’ve been working remotely for about a year and a half. In that time,
I’ve worked from many locations but most of my time has been spent
working from my apartment in Chicago. During this time I’ve tweaked my
environment by building a standing desk, building a keyboard, and
changed my monitor stands. Below is a my desk (click for larger
image).
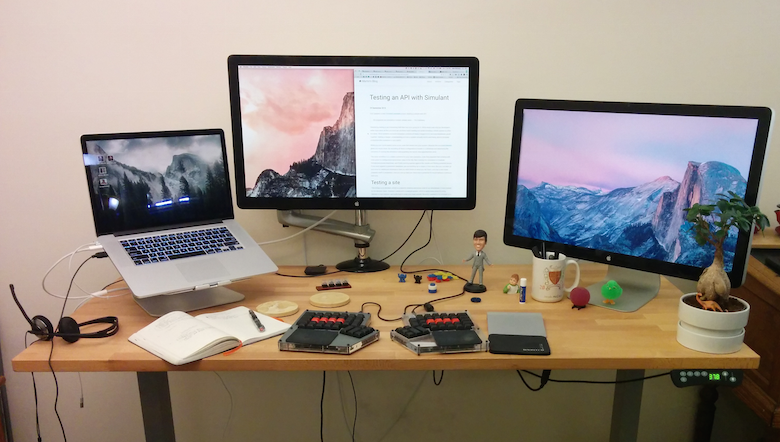
The Desk
I built my own desk using the
Gerton table
top from Ikea and the
S2S Height Adjustable Desk Base
from Ergoprise. I originally received a
defective part from Ergoprise and after a couple emails I was sent a
replacement part. Once I had working parts, attaching the legs to the
table top was straightforward. The desk legs let me adjust the height
of my desk so I can be sitting or standing comfortably.
The Monitors
I have two 27 inch Apple Cinema displays that are usually connected to
a 15 inch MacBook Pro. The picture doesn’t show it, but I actively use
all the monitors.
My laptop is raised by a
mStand Laptop Stand.
While I’m sitting this stand puts the laptop at a comfortable height.
I highly recommend getting one.
The middle monitor, the one I use the most, has had the standard stand
(you can see it in the right monitor) replaced with an
ErgoTech Freedom Arm.
This lets me raise the monitor to a comfortable height when I’m
standing (as seen in this picture). It also allows me to rotate the
monitor vertically, though I have only done that once since installing
it. Installation of the arm wasn’t trivial, but it wasn’t that
difficult.
I’ve been using the arm for four months now and I’m enjoying it. If
you bump the desk the monitor does wobble a bit but I don’t notice it
while I’m typing. I haven’t noticed any slippage; the monitor arm
seems to hold the monitor in place.
I’ve decided against getting a second arm for my other monitor.
Installing the monitor arm renders your monitor non-portable. It
doesn’t happen often, but sometimes I travel and stay at a place for
long enough that I want to bring a large monitor.
The Chair
My desk chair is a Herman Miller Setu. It
is a very comfortable chair that boasts only a single adjustment. You
can only raise or lower it.
I moved to this chair from a Herman Miller
Aeron. The Aeron had been my primary chair
for eight years prior to me buying the Setu.
They are both great chairs. I haven’t missed the extreme amount of
customization the Aeron provides; its actually nice having fewer knobs
to tweak. I also find the Setu more visually appealing. The Aeron is
sort of a giant black monster of a chair; I prefer seeing the
chartreuse Setu in my apartment.
The Keyboard and Mouse
I built my own keyboard. It is an ErgoDox with Cherry MX Blue
key switches and DSA key caps. More details about my build can be found
in an earlier post.
I’ve been using this keyboard for about eight months. It has been rock
solid. This is my first keyboard that has mechanical switches. They
are nice. It feels great typing on this keyboard.
The ErgoDox has six keys for each thumb. I originally thought I’d be
using the thumb clusters a lot but, in practice, I only actively use
two or three keys per thumb.
The ErgoDox also supports having multiple layers. This means that with
the press of a key I can have an entirely different keyboard beneath
my finger tips. It turns out this is another feature I don’t frequently
use. I really only use layers for controlling my music playback
through media keys and for hitting function keys.
If I were going to build a keyboard again I would not use
Cherry MX Blues as the
key switch. They are very satisfying to use but they are loud. You can
hear me type in every room of my one bedroom apartment. When I’m
remote pairing with other developers, they can here me type through my
microphone.
For my mouse I use
Apple’s Magic Trackpad.
I definitely have problems doing precise mouse work (though I rarely
find myself needing this) but I really enjoy the gestures in enables.
I’ve been using one of these trackpads for years now. I really don’t
want to go back to using a mouse.
Other Items
I’m a fan of using pens and paper to keep track of notes. My tools of
choice are
Leuchturm Whitelines
notebook with dotted paper and a
TWSBI 580
fountain pen with a fine nib. I’ve been using fountain pens1 for a
couple years now and find them much more enjoyable to use than other
pen styles. The way you glide across the page is amazing. I usually
have my pen inked with
Noodler’s 54th Massachusetts.
The ink is a beautiful blue black color and very permanent.
No desk is complete without a few fun desk toys. My set of toys
includes a bobble head of myself (this was a gift from a good friend),
a 3d printed
Success Kid,
a
keyboard switch sampler,
a few more 3d printed objects, and some climbing related hand toys.
End
That pretty much covers my physical work space. I’ve tweaked it enough
where I don’t feel like I need to experiment anymore. The monitor arm
is my most recent addition and it really helped bring my environment
to the next level. I think I’ll have a hard time improving my physical
setup.